Coding with Python: The Ultimate Training for Aspiring Developers Bundle
What's Included
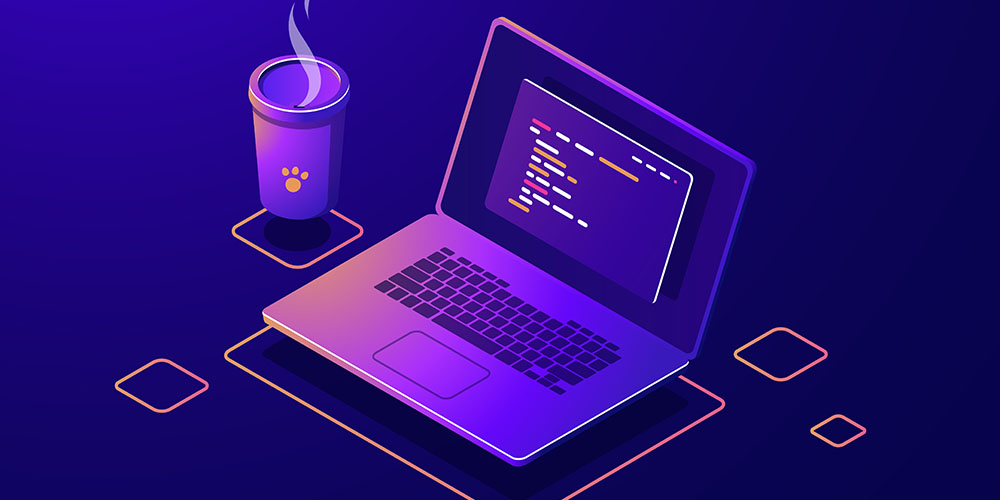
Complete Python Course: Learn Python by Doing
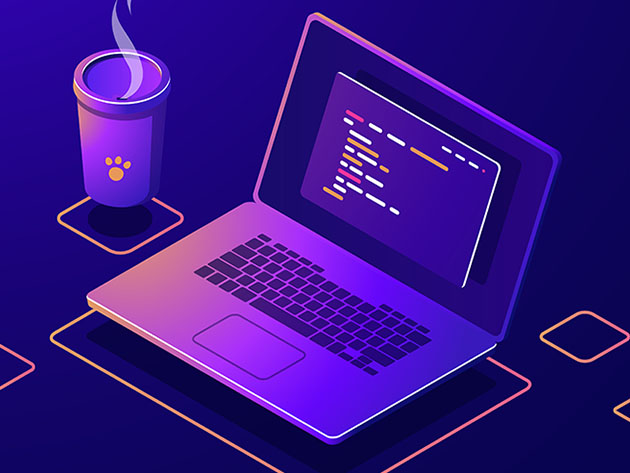
- Experience level required: All levels
- Access 316 lectures & 34 hours of content 24/7
- Length of time users can access this course: Lifetime
Course Curriculum
316 Lessons (34h)
- Your First Program
- Intro to PythonOverview of the course curriculum2:48Our Python coding environment2:28Writing our first code! Variables and printing4:05Community & Support6:00Numbers in Python3:10Calculating the remainder of a division4:09Python strings6:57Python string formatting7:08Getting user input in Python8:07A note about f-strings in code exercisesBooleans and comparisons in Python4:26and & or in Python8:50Lists in Python8:05Tuples in Python5:29Sets in Python2:45Advanced set operations4:51Python dictionaries7:46Length and sum2:47Joining a list2:04
- Python fundamentalsIf statements in Python12:21While loops in Python6:14For loops in Python7:27Destructuring syntax3:19Iterating over dictionaries2:29Break and continue4:11A couple more loop examplesThe else keyword with loops3:48Finding prime numbers with for loops5:35List slicing in Python4:02List comprehension in Python9:02Comprehensions with conditionals6:16Set and dictionary comprehensions5:07The zip function2:42Functions in Python4:10Arguments and parameters10:15Functions and return values in Python9:25Default parameter values7:26Lambda functions in Python6:08First class and higher order functions8:31
- Milestone Project 1Code for this sectionInstalling Python in your computer2:18Installing PyCharm in your computer2:56Creating our first PyCharm project5:45Setting up PyCharm font and display settings2:43Milestone Project briefCoding our application's menu in Python13:19Adding new movies to our application9:37Showing the user their movies6:58Finding movies and retrieving their details12:48
- Object-Oriented Programming with PythonIntro to Object-Oriented Programming with Python17:02More about classes and objects9:17Parameter naming in Python3:54Magic methods in Python12:57Inheritance in Python8:45The @property decorator4:19@classmethod and @staticmethod in Python7:27More @classmethod and @staticmethod examples12:06
- Errors in PythonCode samples for this sectionIntro to errors in Python13:13Built in errors in Python17:58Raising errors in Python10:58Creating our own errors in Python13:42Dealing with Python errors9:00The on success block and re-raising exceptions11:21Handling those pesky user errors!14:55Debugging with Pycharm16:37Further reading
- Files in PythonCode for this sectionFiles in Python10:55Python Exercise: copying files20:38CSV files with Python9:35How to use the csv module to read and write CSV files more easilyJSON files with Python17:33Using the with syntax in Python5:01Importing our own files10:28Python relative imports: children15:18Python relative imports: parents5:44Import errors and running as a Python script5:26Further reading
- Databases in Python & Milestone Project 2Code for this sectionIntro to Milestone Project 25:53Milestone Project 2 BriefMilestone Project with lists17:52Storing books in files19:30Using JSON instead of CSV9:58Intro to databases with Python7:03Using SQLite in Python2:37Some database jargon4:08Creating our books table using Python5:49Inserting books using Python6:19SELECT examples3:12Getting all our books6:30UPDATE and DELETE2:14Filtering with WHERE4:59Finishing the Milestone Project7:46Ordering and limiting2:37Developing our context manager in Python10:11Errors in context managers6:55Further reading
- Type hinting in PythonFurther reading1:44Typing in Python12:10
- Advanced built-in functions in PythonGenerators in Python12:39Python generator classes and iterators8:15The filter() function in Python8:43Iterables in Python7:48The map() function in Python5:39any() and all() in Python4:59The enumerate() function4:19So what other things evaluate to True or False?Further reading
- Advanced Python DevelopmentMutability in Python11:49Argument mutability in Python12:16Default values for parameters5:03Mutable default arguments (bad idea)6:38Argument unpacking in Python11:16Queues in Python3:20Some interesting Python collections25:55Timezones8:46Dates and time in Python12:46Timing your code with Python12:32Regular expressions8:28Regex examples13:36Regex in Python13:07Introduction to logging in Python10:35Logging to a file and other features4:16The built-in itertools moduleFurther reading
- Web Scraping with PythonCode for this sessionUnderstanding HTML with BeautifulSoup18:44More complex HTML parsing22:18Structuring our parsing program better5:40Splitting HTML locators out of our Python class4:03Understanding HTML with the browser6:35Scraping our first website with Python6:42Milestone Project 3: A Quote Scraper4:13Quotes Project 2: Structuring a scraping app in Python2:41Quotes Project 3: Getting our locators5:09Quotes Project 4: Crafting our quote parser4:51Quotes Project 5: The quotes page4:30Quotes Project 6: Recap of the project3:54Milestone Project 4: A Book Scraper + application5:01Books Project 2: Recap of HTML locators4:53Books Project 3: Creating locators in Python4:26Books Project 4: Creating our page3:27Books Project 5: Creating our book parser11:01Books Project 6: Writing our app file3:55Books Project 7: Sorting the books8:50Books Project 8: Constructing our menu6:02ASIDE: The best way to write user menus1:28Books Project 9: Getting multiple pages2:37Books Project 10: Multiple pages in Python5:26Books Project 11: Getting the page count in Python6:33Books Project 12: Adding logging to our Python project20:28A word on scraping pages with JavaScript3:14A note on scraping and robots.txt
- Browser automation with SeleniumIntroduction to this section1:38Code for this sectionReview of our quotes scraping code5:01Downloading chromedriver2:00Using Chrome in our scraping code6:26Our new page locators3:44Interacting with dropdowns4:07Selecting tags5:17Searching for quotes2:17Encapsulating logic more simply5:03Adding some error handling1:39Implicit and explicit waits in Selenium6:49Adding waits to our program code7:04
- Asynchronous Python DevelopmentCode samples for this sectionA glossary of terms used in concurrencyThe Dining Philosophers Problem7:38Processes and threads9:24The Python GIL10:30Example: threads in Python12:40Using Python concurrent.futures: the ThreadPoolExecutor3:08Don't kill threads!1:48Multiprocessing in Python7:24Using Python concurrent.futures: the ProcessPoolExecutor2:15Dealing with shared state in threads8:31Queuing in threads with shared state12:25Using Python generators instead of threads4:50Our first single-threaded task scheduler in Python6:04Yielding from another iterator in Python5:36Receiving data through yield9:34The async and await keywords6:10Watch these talks for more explanations and examples!1:52Our asynchronous scraper4:42Making our first async request in Python14:49Getting multiple pages efficiently10:23Using async_timeout for security1:46Turning our book scraping project async10:16A note on HTTPS with Python and Mac OS X3:12
- Python on the console and managing project dependenciesRunning Python in the console8:49Terminal video: running Python5:50Terminal video: what is a virtualenv?10:43Terminal video: navigating the terminal and using virtualenv9:49Terminal video: using Pipenv9:38Terminal video: Pipenv and virtualenv4:05Summary e-book of using Pipenv
- Web development with FlaskSetting up our project with Pipenv5:42Code samples for this sectionOur first Flask endpoint7:37Returning information with Flask and Python4:55Rendering HTML with Flask and Python6:24Error pages and Jinja2 inheritance11:39Rendering forms with Flask and Python14:21Accessing POST form data with Flask4:48Putting our form in a single endpoint3:02Using Jinja2 for loops to create a nicer homepage7:57Adding navigation to our website4:54
- Interacting with APIs with PythonCode for this sectionSigning up to OpenExchangeRates1:41Getting all exchange rates from the API5:30Creating a currency exchange library7:25Caching functions with functools4:49TTL caches with cachetools2:04
- Decorators in PythonA simple decorator in Python6:07Using a @syntax3:23Functools wraps in Python1:55Decorating functions with parameters4:56Decorators with parameters9:50Functions that accept multiple arguments5:29Generic decorators for any function3:54Multiple decorators for one function?
- Advanced Object-Oriented ProgrammingIntro to multiple inheritance with Python13:23Intro to ABCs in Python8:06The usefulness of ABCs3:20The relationship between ABCs and interfaces6:49The property setter in Python11:08Pythonic vs. Non-Pythonic
- GUI Development with TkinterCode for this sectionSetting up Tkinter2:47Hello world in Tkinter7:37Labels and fields5:22Packing components11:57Using frames for different layouts3:41Starting our text editor project0:56Tkinter notebooks and creating files3:32Adding a menu to our application4:11Saving files to disk7:59Opening files4:19Binding shortcuts in Tkinter4:42Checking our tabs for unsaved changes8:17Confirming exit with unsaved changes4:24Closing individual tabs5:21Adding another menu1:55Adding a permanent scrollbar to our text area4:22
- Unit testing with PythonIntroduction to this section2:53Code for this sectionTesting functions7:26Testing for errors2:37Testing our multiplication function9:08Writing a printer class for testing1:59Testing classes4:44More Printer tests9:26Testing external libraries9:53Conclusion of this section0:58
- Algorithms and Data StructuresPresentation: queues, stacks, and complexity9:40A conference talk about Big-OPresentation: binary search6:47Presentation: binary trees5:53Presentation: traversal of binary trees7:12Presentation: adding elements to a binary tree7:00Adding elements to a binary tree in Python10:58Recursion and inorder traversal in Python14:35Finding nodes in a tree with Python3:21How do you delete nodes from a binary tree?9:29Deleting nodes in code with Python14:10Deleting nodes with two children in code18:44Testing our binary tree!2:37
- Python librariesPython libraries overview16:35Using pylint12:40Using yapf7:42Sending e-mails with smtplib7:35Sending e-mails with Mailgun9:53Creating a re-usable Mailgun library7:11Sneak peek: my IDE setup!9:30
- Python Reference / RefresherIntroduction to this section1:01Variables in Python8:26String formatting in Python6:26Getting user input5:16Lists, tuples, and sets6:31Advanced set operations4:39Booleans in Python4:59If statements in Python8:17The in keyword2:02If statements with the 'in' keyword8:18Loops in Python11:07List comprehensions7:24Dictionaries8:31Destructuring variables8:28Functions in Python10:41Function arguments and parameters7:40Default parameter values3:54Functions returning values7:19Lambda functions in Python7:52Dictionary comprehensions4:01Unpacking arguments10:24Unpacking keyword arguments8:44Object-Oriented Programming in Python15:52Magic methods: str and repr 6:25Class methods and static methods14:03Class inheritance8:32Class composition6:08Type hinting5:08How imports work in Python9:33Relative imports in Python8:52Errors in Python12:47Custom error classes5:04First-class functions7:52Simple decorators in Python7:12The 'at' syntax for decorators3:32Decorating functions with parameters2:24Decorators with parameters4:50Mutability in Python6:03Mutable default parameters (and why they're a bad idea)4:27
Complete Python Course: Learn Python by Doing
Jose studied Applied Computing at the University of Dundee, one of the most prestigious universities for computing-related courses, and he was offered the chance to participate in their computing scholarships due to academic achievement.
He has worked for Eseye, an M2M company, as an intern doing mainly backend developing, writing PHP scripts, and programming Zenoss ZenPacks, and currently works for Skyscanner, one of Scotland's largest technology companies, programming mainly in Python and web languages.
Rob Percival | Web Developer | Teacher
Description
Demand for Python is booming in the job market and it is a skill that can help you enter some of the most exciting industries, including data science, web applications, home automation and many more. Python is one of the "most loved” and “most wanted” programming languages according to recent industry surveys. If people are not using Python already, they want to start using Python. With 34 hours of instruction, this course will take you from beginner to expert in Python. It will cover major topics including Object-Oriented Programming, Web Scraping, GUI development, and more. This course will make it easy for you to learn Python and get ahead of your competition.
6,067 positive ratings from 25,596 students enrolled
- Access 316 lectures & 34 hours of content 24/7
- Get a broader & deeper experience in Python
- Start at zero & become an expert whilst learning all about the inner workings of Python
- Learn how to write professional Python code like a professional Python developer
- Develop a long-lasting love for Python & programming by creating good programming habits
- Explore the wider possibilities of what you can do w/ Python, including databases, web development & web scraping
- Become job-ready by learning about best practices, Selenium, unit testing, & all of the major Python topics
"Jose Salvatierra is wonderful teacher. His command on the subject is awesome and he gives complete effort and shows complete dedication to make his student understand thoroughly. I recommend this course for sure." – Munish Kumar Sharma
Specs
Important Details
- Length of time users can access this course: lifetime
- Access options: web & mobile streaming
- Certification of completion included
- Redemption deadline: redeem your code within 30 days of purchase
- Updates included
- Experience level required: all levels
- Have questions on how digital purchases work? Learn more here
Requirements
- Any device with basic specifications
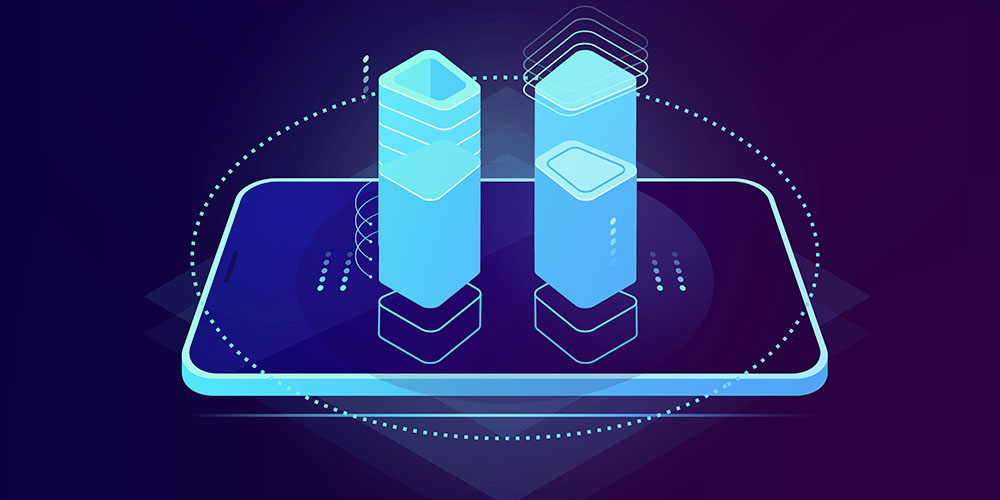
REST APIs with Flask & Python
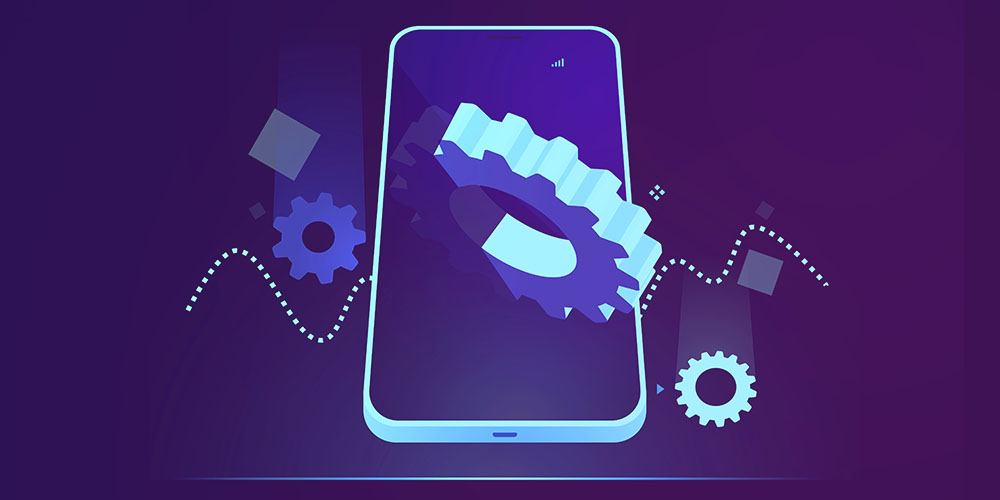
Advanced REST APIs with Flask & Python
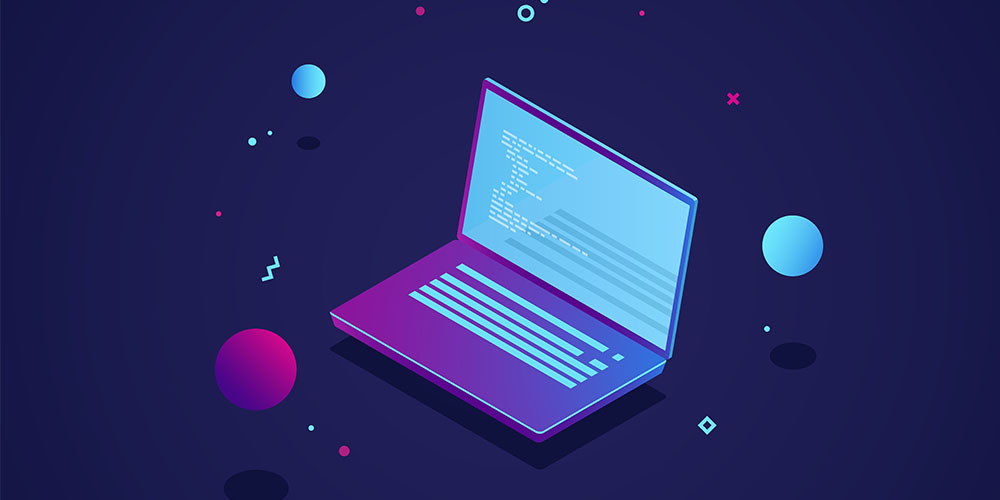
Automated Software Testing with Python
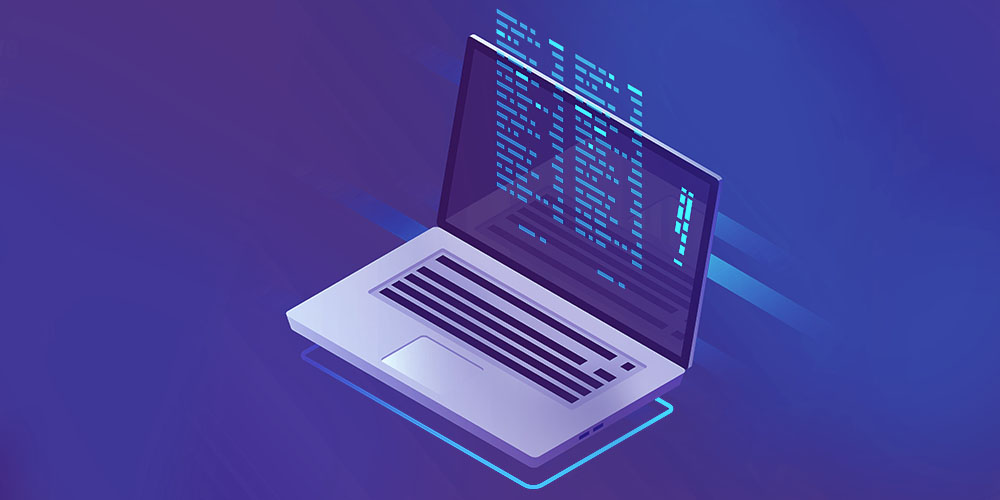
Git by Example
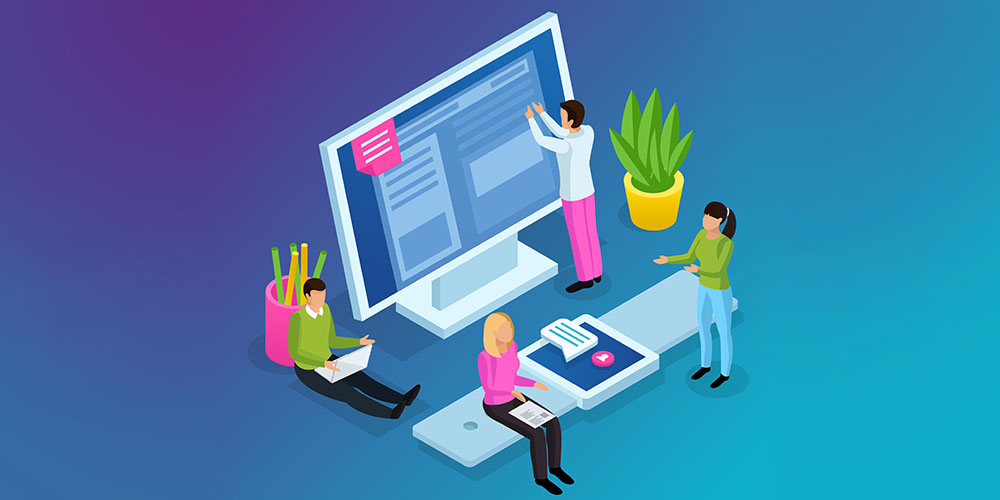
Complete Python Web Course: Build 8 Python Web Apps
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.
Mansi Adhiya
Very informative and makes learning easy for someone like me who is very new to Phython and coding in general....Highly recommend!!!
Oliver Anderson
Clear and simple presentation of easy to understand and applicable data! Very pleasant and enjoyable to follow. Thank You!